Interactions with Gameplay Effects
There are several functions that an Attribute Set can override to deal with the way an Attribute responds when a Gameplay Effect attempts to modify it.
Most of this API is exposed to Blueprints (PostGameplayEffectExecute, Pre/PostAttributeChange, ...).
PreAttributeChange / PreAttributeBaseChange
They serve a similar purpose and share the same method signature.
One is invoked when the Current Value is modified, the other when the Base Value is changed.
To learn more about BaseValue vs CurrentValue, check out 4.3.2
part of the most excellent GASDocumentation on GitHub (opens in a new tab).
Permanent changes to the BaseValue come from Instant GameplayEffects whereas Duration and Infinite GameplayEffects change the CurrentValue. Periodic GameplayEffects are treated like instant GameplayEffects and change the BaseValue.
PreAttributeChange
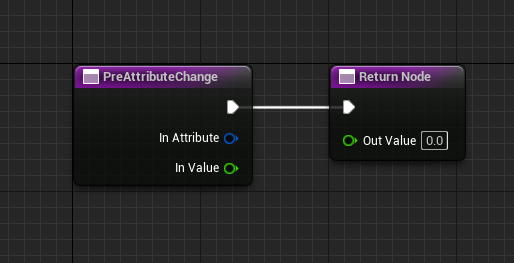
Method description
Called just before any modification happens to an attribute. This is lower level than PreAttributeModify/PostAttribute modify.
There is no additional context provided here since anything can trigger this. Executed effects, duration based effects, effects being removed, immunity being applied, stacking rules changing, etc.
This function is meant to enforce things like "Health = Clamp(Health, 0, MaxHealth)" and NOT things like "trigger this extra thing if damage is applied, etc".
OutValue is a mutable reference so you are able to clamp the newly applied value as well
Note When overriding in BP, OutValue is the return value of the overriden BP function, make sure to pass in the new value. Typical implementation should simply return the input value parameter (or a clamped value based on InValue).
Parameters
Name | Type | Description |
---|---|---|
InAttribute | const FGameplayAttribute& | The Gameplay Attribute whose current (or final) value is about to change |
InValue | float | Original value for the Gameplay Attribute |
OutValue | float | Return value of the function which represents the new current value for the Gameplay Attribute |
PreAttributeBaseChange
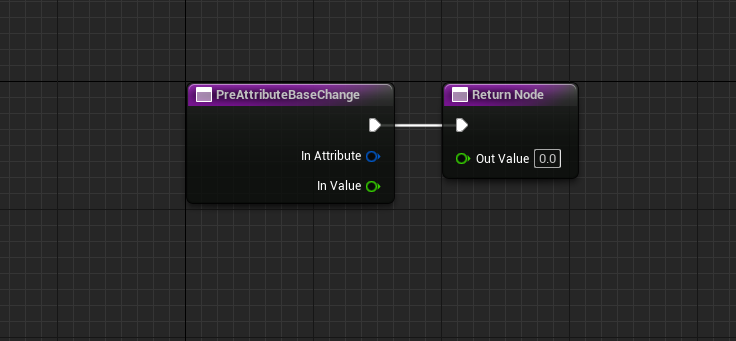
Method description
This is called just before any modification happens to an attribute's base value.
This function should enforce clamping (presuming you wish to clamp the base value along with the final value in PreAttributeChange)
This function should NOT invoke gameplay related events or callbacks. Do those in PreAttributeChange() which will be called prior to the final value of the attribute actually changing.
Parameters
Name | Type | Description |
---|---|---|
InAttribute | const FGameplayAttribute& | The Gameplay Attribute whose base value is about to change |
InValue | float | Original value for the Gameplay Attribute |
OutValue | float | Return value of the function which represents the new base value for the Gameplay Attribute |
PostAttributeChange / PostAttributeBaseChange
Similar pattern as previous section about Pre-hooks, they share similar pattern and method signature.
The only difference between the two is that PostAttributeBaseChange
is const, whereas PostAttributeChange
is not (making the latter an implementable Event - as opposed to a overriden BP function).
PostAttributeChange
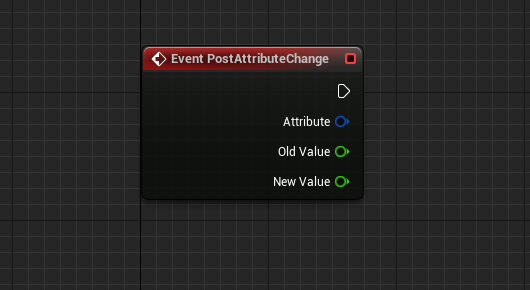
Method description
Called just after any modification happens to an attribute.
Parameters
Name | Type | Description |
---|---|---|
InAttribute | const FGameplayAttribute& | The Gameplay Attribute whose value has been changed |
OldValue | float | Original value for the Gameplay Attribute, before PreAttributeChange event and actual modification happened |
NewValue | float | New value for the Gameplay Attribute, after PreAttributeChange event and modification happened |
PostAttributeBaseChange
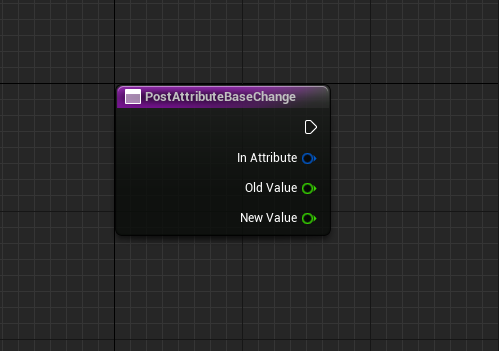
Method description
Called just after any modification happens to an attribute's base value right and after aggregation (when an attribute aggregator exists).
Parameters
Name | Type | Description |
---|---|---|
InAttribute | const FGameplayAttribute& | The Gameplay Attribute whose base value has been changed |
OldValue | float | Original value for the Gameplay Attribute, before PreAttributeBaseChange event and actual modification happened |
NewValue | float | New value for the Gameplay Attribute, after PreAttributeBaseChange event and modification |
PreGameplayEffectExecute
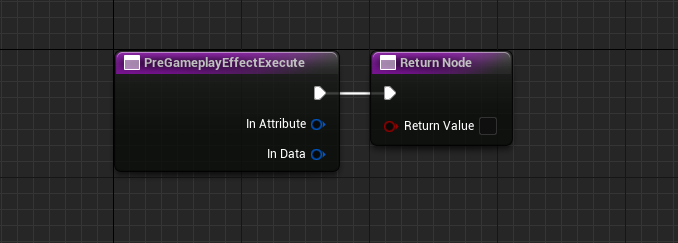
Method description
Called just before modifying the value of an attribute. AttributeSet can make additional modifications here.
Return true to continue, or false to throw out the modification
Note this is only called during an 'execute'. E.g., a modification to the 'base value' of an attribute. It is not called during an application of a GameplayEffect, such as a 5 second +10 movement speed buff.
This should apply 'gamewide' rules. Such as clamping Health to MaxHealth or granting +3 health for every point of strength, etc PreGameplayEffectExecute can return false to 'throw out' this modification.
Note: Be extra careful when overriding this function in BP, make sure to return true, otherwise the Gameplay Effects won't apply anymore.
Since the default behavior for the editor is to implement the method with return value set to false, this is something to be wary off.
Parameters
Name | Type | Description |
---|---|---|
InAttribute | const FGameplayAttribute& | The Gameplay Attribute whose base value has been changed |
InData | const FGBAAttributeSetExecutionData& | Payload with information extracted from FGameplayEffectModCallbackData, the Source / Target actor, Ability System Component, Controllers, Effect Context, Specs and Source Tags, Magnitude and Delta values, etc. |
Return value
Return true to continue, or false to throw out the modification.
PostGameplayEffectExecute
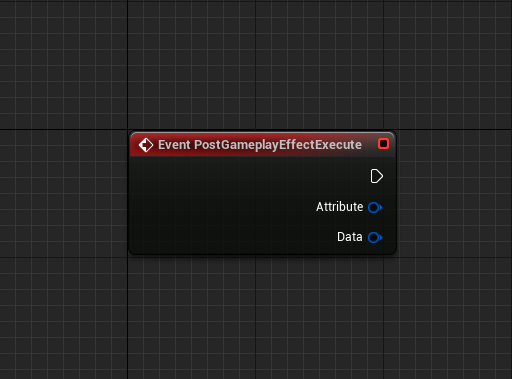
Method description
Called just after a GameplayEffect is executed to modify the base value of an attribute. No more changes can be made.
Note this is only called during an 'execute'. E.g., a modification to the 'base value' of an attribute. It is not called during an application of a GameplayEffect, such as a 5 second +10 movement speed buff.
This should apply 'gamewide' rules. Such as clamping Health to MaxHealth or granting +3 health for every point of strength, etc
Parameters
Name | Type | Description |
---|---|---|
Attribute | const FGameplayAttribute& | The Gameplay Attribute whose value has been changed by a Gameplay Effect |
InData | const FGBAAttributeSetExecutionData& | Payload with information extracted from FGameplayEffectModCallbackData, the Source / Target actor, Ability System Component, Controllers, Effect Context, Specs and Source Tags, Magnitude and Delta values, etc. |
struct FGBAAttributeSetExecutionData
Structure holding various information to deal with AttributeSet lifecycle events (such as Pre/PostGameplayEffectExecute), extracting info from FGameplayEffectModCallbackData.
This struct is used as an input parameter for some of the AttributeSet BP exposed event / methods that are using FGameplayEffectModCallbackData
in native code (PreGameplayEffectExecute and PostGameplayEffectExecute)
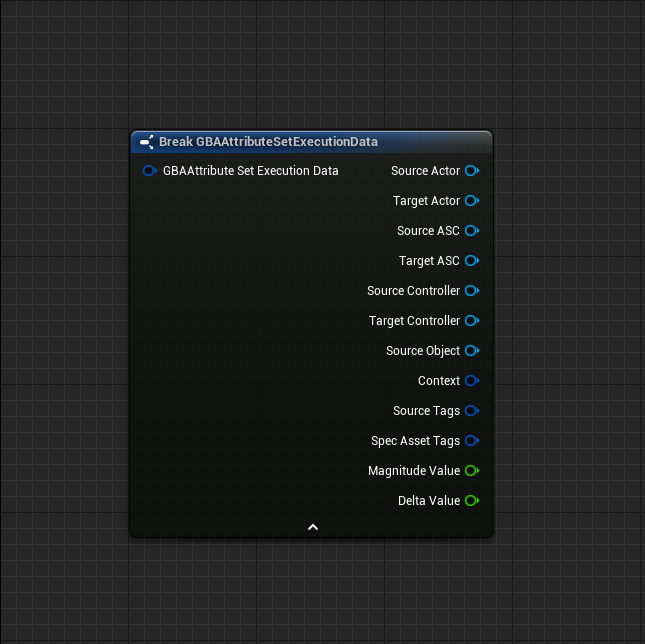